Avoid Vertical Limits in Microservice Architectures
The microservice architecture allows us to partition an application into tiny sub-applications, which are easy to maintain and to deploy. This pattern is already widely adopted to implement backend systems. But the frontend is usually still one large application, at least when it comes to deployment. This article describes some thoughts on how to address this problem.The microservice architecture is en vogue, everybody seems to know all about it, and feels like having to spread the truth. Including me ;-) But honestly, we are just about to learn how to cope with this kind of architecture. I guess we are like a kid that just managed to make some first steps, when we suddenly try to start running… that’s usually the moment when you fall over your own feet. Microservices are no free lunch, it definitely has its price (that’s the part we have already learned). For developers it feels uncomfortable, since instead of developing one application, they have to deal with dozens or hundreds. Usually the microservice-believers are the people who drive and maintain an application over a long period of time; those poor chaps that know the pain of change. And that is were their trust – or better: hope – in microservices comes from.
So what is this article about? I’m currently working in a web project for a customer, where we are using Spring Boot to create a microservice-based backend. We heavily use the Job DSL to drive our CI pipeline, and also all kind of configuration and tooling. And the front end is a single page application built with AngularJS. So far, so good, but there is a catch. One goal of this microservice idea is to have independent development teams, that drive features within their own development cycle. In the backend this is feasible due to the microservice architecture. But the front end is still one large application. Angular allows to partition the application into modules, but after all it is assembled to one application, so this is a single point of integration. Let me explain that point using a practical example. It is a stupid-simple web shop, stripped down to the bones. It is just a catalog of items, which we can put into a shopping cart:
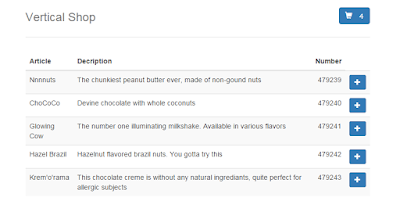
I have prepared the example as a Spring Boot based application in the backed, with some AngularJS in the frontend. You will find the code on GitHub, the version we start with, is on the branch one-ui. All projects have maven and gradle builds, the readme will provide you enough information to run the application.
Just some note before we start: I’m not a web developer. In the last 20 years I have build – beside backend systems – various UIs in Motif, QT, Java AWT, Swing and SWT. But I have never done web frontends, there has never been the chance or need for that. So please be merciful if my angular and javascript looks rather… er, surprising to you ;-)The architecture of our stupid simple web shop looks like this:
The browser accesses the application via a router, which limits access to the backend, helps with the CORS problem, and may also perform some security checks. The web-server hosts the AngularJS application and the assets. The AngularJS application itself communicates with the API-Gateway, which encapsulates the microservices in the backend, and provides services and data optimized for the UI. The API-Gateway pattern is often used in order to not to bloat the microservices with functionality where it not belongs to. We will see its advantages later on. The gateway talks to the backend microservice, which then perform their dedicated functionality using their own isolated databases (the databases has been omitted for simplicity in the example code).
So far nothing new, what’s the point? Well, what is that microservice idea all about? Ease of change. Make small changes easy to apply and deploy. Minimize the risk of change by having to deploy only a small part of the application instead of a large one-size-fits-all application. In the backend this is now widely adopted. The microservices are tiny, and easy to maintain. As long as their interfaces to other services remain (compatible), we may replace them with a new version in a running production environment, without affecting the others. And – if done right – without downtime. But what about the frontend? The frontend is mostly still one large application. If we make a small change to the UI, we have to build and redeploy the whole thing. Even worse, since it is one application, bug fixes and features are often developed in parallel by large teams, which makes it difficult to release minor changes separately. At last, that’s what the microservice story is all about: Dividing your application into small sub-applications which can be developed and deployed separately, giving each its own lifecycle. And consequently, this pattern should be applied to the complete application, from database via services to the frontend. But currently, our microservice architecture thinking ends at the UI, and that’s what I call a vertical limit.
We investigated how other people are dealing with this situation, and – no wonder – there were a lot of complaints about the same problem. But surprisingly, most advices to address this were to use the API-Gateway… er, but this does not solve the problem?!? So let’s think about it: we are dividing our backend into fine grained microservices in order to cope with changes. Consequently, we should exactly the same in UI. We could partition our UI into components, where features make up the logical boundaries. Let’s take our silly vertical shop example to exercise this. We separate this UI at least into two logical components: the catalog showing all available items, and the shopping cart. Angular provides a mechanism to build components: directives! The cart and the catalog are already encapsulated in angular directives (what a coincidence ;-). So what if we put those parts each on its own web-server? Let’s see how our architecture would look like:
Hmm, obviously the API gateway is still a common denominator. The gateway’s job is to abstract from the backend microservice and to serve the UI in the best suited way. So consequently, we should divide the gateway also. But since it so closely related to the UI, we are packaging ‘em both into one service in our example. Let’s do so, you will find the code for this version on the branch ui-components. Now the architecture looks like this:
Hey wait! I’ve had look at your UI code. It’s not just the directives, there are also angular services. And the add-button in the catalog directive is effectively calling addItem() on the cart-service. That’s quite true. But is that a problem? In our microservice backend, services are calling other services also. This is not a problem, as long as the service’ API doesn’t change, resp. remains compatible. The same holds on the javascript side. As long as our API doesn’t change (remains compatible), new service rolllouts and internal changes are not a problem. But we have to design these interfaces between components wisely: (angular) services may be used by other components, so we have to be careful with changes. Another way of communication between components is broadcasting events. Actually the cart component is firing an event every time the cart changes, in order to give other components a chance to react on that. So we have to be cautious with changes to events also. New resp. more data is not a problem, removing or renaming existing properties is. So we simply put all functionality dealing with the cart on the cart web-server, and the catalog stuff on the catalog web-server… including their dedicated directives, services, assets a whatsoever. Our communication looks like this:
Big words and bloated architecture. It ain’t worth it! You think so? Let’s make a small change to our application and examine its impact: Our customer does not like our shopping cart: “It just shows each items article number, and its amount. That’s not quite helpful. The user needs the article’s name…its price…and the sum of all items in the cart.”

Well, yeah, she’s right. So let’s fix that. But the cart microservice does not provide more information. Article names and prices are the catalog service’ domain. So the cart service could call the catalog service and enrich its data? But that’s not the cart’s domain, it should not have to know about that. No, providing the data in a way appropriate for the UI is the job of the API gateway (finally it pays ;-). So instead of delegating all calls to the cart service, the cart’s API gateway merges the data from the cart with the catalog data to provide cart items with article names and prices:
Now we simply adapt the UI code of the cart in order to use and visualize this additional data. You will find the source code of this final version on the master. All changes we made are isolated in the cart UI resp. its API gateway, so we only have to redeploy this single server. Let’s do so. And if we now reload our application, tada:

So, we made a change to the shopping cart without affecting the remaining application. Without redeploying the complete UI. All the advantages we take the microservice-burden on our shoulders for, now pays in the front end also. Just treat your front end as a union of distinct features. In agile development it is all about features resp. stories, and now we we are able develop and release features in its own lifecycle. Hey wait, the index.html still assembles the cart and the catalog to a single page. So there is still a single point of integration! Yep, you’re right. This is still one UI, but we have componentized it. Once a component is referenced in a page, that’s it. Any further changes to internals of that component does not affect the hosting page. Our microservices are also referenced by other parties, so this is quite similar.
The point is to avoid vertical limits in this kind of architecture. We have to separate our application into small sub-applications in all layers, namely persistence, service backend and frontend. In the backend this cut is often domain-driven, and in the front end we can use features to partition our application. For sure there are better ways and technologies to implement that, but I guess this is a step into the right direction.
We learn from failure, not from success!
Bram Stoker
1) This was poor attempt to avoid the word monolith, which is – at the time of writing – mistakenly flavored with a bad smack.
The post shared was very nice.
ReplyDeleteMicroservices Online Training
Needed to compose you a very little word to thank you yet again regarding the nice suggestions you’ve contributed here.
ReplyDeleteThank you a lot for providing individuals with a very spectacular possibility to read critical reviews from this site.
Visit Us:http://www.zerobugacademy.com/angularjs-training-in-chennai
Well written Blog, I really enjoy reading your blog. this info will be helpful for me. Thanks for sharing.
ReplyDeleteOracle Training in Chennai | Certification | Online Training Course | Oracle Training in Bangalore | Certification | Online Training Course | Oracle Training in Hyderabad | Certification | Online Training Course | Oracle Training in Online | Oracle Certification Online Training Course | Hadoop Training in Chennai | Certification | Big Data Online Training Course
Awesome blog. I enjoyed reading your articles. This is truly a great read for me. I have bookmarked it and I am looking forward to reading new articles. Keep up the good work!
ReplyDeleteSalesforce Training in Chennai
Salesforce Online Training in Chennai
Salesforce Training in Bangalore
Salesforce Training in Hyderabad
Salesforce training in ameerpet
Salesforce Training in Pune
Salesforce Online Training
Salesforce Training
This comment has been removed by a blog administrator.
ReplyDelete